Build a Philosophy Quote Generator with Vector Search and Astra DB (Part 3)
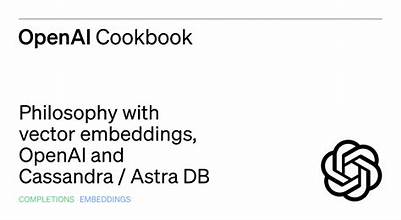
Introduction to Building a Philosophy Quote Generator with Vector Search and Astra DB (Part 3)
In this third part of our series on creating a philosophy quote generator, we will continue exploring how to build a philosophy quote generator with vector search and Astra DB (part 3). This time, we will dive deeper into the technical aspects of integrating vector search with a NoSQL database like Astra DB, focusing on the practical steps required to ensure that your application functions efficiently and effectively. Whether you’re a developer or someone interested in building a meaningful and scalable quote generator, this guide will help you understand the intricacies of vector search and its integration with Astra DB to retrieve the perfect philosophical quote.
Table of Contents
What is Vector Search and Why Use It for a Quote Generator?
Before we get into the specifics of how to build a philosophy quote generator with vector search and Astra DB (part 3), it’s important to first understand vector search and its relevance to the project. Vector search involves representing words, phrases, or entire sentences as mathematical vectors, where each vector is a point in a high-dimensional space. This allows for similarity comparison between words or sentences based on their meaning rather than exact wording. In the context of a quote generator, vector search can be used to find quotes that are semantically similar to a given input, making the results more contextually relevant.
For example, instead of just searching for the exact phrase typed by a user, a vector search can return quotes that convey similar sentiments even if they do not use the same words. This is especially valuable for philosophical quotes, where many different authors may express similar thoughts but in distinct ways.
Incorporating vector search into your philosophy quote generator allows for smarter, more intuitive search results and an enhanced user experience.
Getting Started with Astra DB
Astra DB is a cloud-native database built on Apache Cassandra, which provides highly scalable and highly available NoSQL database services. It’s an excellent choice for applications like a philosophy quote generator because of its flexibility, scalability, and ease of integration with cloud-based solutions.
To build a philosophy quote generator with vector search and Astra DB (part 3), you need to first set up your Astra DB instance. Once your instance is running, you will be able to store large volumes of data such as the philosophical quotes you wish to search through, as well as metadata related to those quotes, such as the author and category of the quote.
In Part 3 of this series, we will look at how to integrate Astra DB into your application and store vectors representing each quote, making use of vector search to retrieve the most relevant quotes based on semantic similarity.
Step 1: Preparing Your Data

Before we jump into coding and integrating the database, it’s essential to prepare the data that will populate your quote generator. build a philosophy quote generator with vector search and Astra DB (part 3) You’ll need a collection of philosophical quotes that you will store in Astra DB.
Here’s what your data should include:
- Quote Text: The actual text of the philosophical quote.
- Author: The author who is associated with the quote.
- Category/Theme: A category or theme to which the quote belongs (e.g., existentialism, ethics, etc.).
- Vector Representation: A vector representation of the quote, generated using natural language processing (NLP) techniques.
To generate the vector representations of your quotes, you can use popular NLP models like GPT, BERT, or Sentence-BERT. Build a Philosophy Quote Generator with Vector Search and Astra DB (Part 3) These models will convert each quote into a numerical representation (vector) that captures the semantic meaning of the quote.
Once the data is prepared, you can store it in Astra DB using tables designed to hold both the text of the quotes and their associated vectors.
Step 2: Creating the Data Model in Astra DB
In Astra DB, you will create a schema that reflects the structure of the data we just discussed. Below is an example schema for your quote generator.
CREATE KEYSPACE quotes WITH REPLICATION = {'class' : 'SimpleStrategy', 'replication_factor' : 3};
CREATE TABLE quotes.quotes_data (
quote_id UUID PRIMARY KEY,
quote_text TEXT,
author TEXT,
category TEXT,
vector_value LIST<FLOAT>
);
In this schema:
- quote_id: A unique identifier for each quote (UUID).
- quote_text: The actual text of the quote.
- author: The author of the quote.
- category: The thematic category the quote falls under.
- vector_value: A list of floating-point numbers representing the vector for the quote.
Once your schema is set up, build a philosophy quote generator with vector search and Astra DB (part 3) you can start inserting your quotes into the database.
Step 3: Storing Vector Representations in Astra DB
Now that your schema is ready, it’s time to store the vector representations of your quotes. As mentioned earlier, you can generate these vectors using a pre-trained NLP model. In this case, let’s assume you’re using a Python environment with the sentence-transformers
library to create these vectors.
Here’s an example of how you would generate the vector for a single quote and insert it into the Astra DB:
from sentence_transformers import SentenceTransformer
import cassandra
from cassandra.cluster import Cluster
# Load the model
model = SentenceTransformer('all-MiniLM-L6-v2')
# Example quote
quote_text = "The only true wisdom is in knowing you know nothing."
author = "Socrates"
category = "Wisdom"
# Generate the vector representation of the quote
vector = model.encode(quote_text).tolist()
# Connect to Astra DB
cluster = Cluster(['<your_astra_db_host>'])
session = cluster.connect('<your_keyspace>')
# Insert quote data into Astra DB
session.execute("""
INSERT INTO quotes.quotes_data (quote_id, quote_text, author, category, vector_value)
VALUES (uuid(), %s, %s, %s, %s)
""", (quote_text, author, category, vector))
This code connects to your Astra DB instance and inserts a quote along with its vector representation. With this setup, build a philosophy quote generator with vector search and Astra DB (part 3) your quotes are ready for vector-based search!
Step 4: Implementing Vector Search with Astra DB
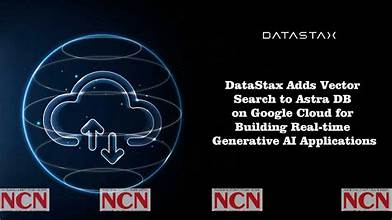
Now, the fun part begins! To build a philosophy quote generator with vector search and Astra DB (part 3), you need to implement vector search functionality that can compare the query vector with the stored vectors in your database.
There are a few ways to implement vector search, but since Astra DB is a NoSQL database, it doesn’t natively support vector search as relational databases do. build a philosophy quote generator with vector search and Astra DB (part 3) However, there are workarounds.
One common method is to calculate the cosine similarity between the query vector and stored vectors. The cosine similarity measures the cosine of the angle between two vectors. The smaller the angle (i.e., the higher the cosine similarity), the more similar the vectors are.
Here’s how you might perform the vector search:
import numpy as np
from cassandra.cluster import Cluster
def cosine_similarity(v1, v2):
return np.dot(v1, v2) / (np.linalg.norm(v1) * np.linalg.norm(v2))
# Connect to Astra DB
cluster = Cluster(['<your_astra_db_host>'])
session = cluster.connect('<your_keyspace>')
# Example query
query = "What is true wisdom?"
# Generate the vector for the query
query_vector = model.encode(query).tolist()
# Fetch all quote vectors from the database
rows = session.execute("SELECT quote_id, vector_value FROM quotes.quotes_data")
# Find the most similar quote
best_similarity = -1
best_quote = None
for row in rows:
stored_vector = np.array(row.vector_value)
similarity = cosine_similarity(query_vector, stored_vector)
if similarity > best_similarity:
best_similarity = similarity
best_quote = row.quote_id
# Retrieve the best matching quote
result = session.execute("SELECT quote_text, author FROM quotes.quotes_data WHERE quote_id = %s", (best_quote,))
print(result[0].quote_text, result[0].author)
This code fetches all the vectors from your Astra DB instance, build a philosophy quote generator with vector search and Astra DB (part 3) compares each one with the query vector, and returns the quote with the highest cosine similarity.
Step 5: Enhancing the Search and Adding Features
To further enhance the build a philosophy quote generator with vector search and Astra DB (part 3), you can add features such as:
- Filtering by Category: You can allow users to filter quotes by categories like “Wisdom,” “Love,” or “Ethics,” providing more targeted results.
- Ranking Results: Instead of returning just the best result, rank the top N most similar quotes and return them as a list to the user.
- Caching: To speed up search times, consider caching the most popular or most recent queries.
- User Feedback: Incorporate a feedback loop where users can rate the relevance of the quotes, improving the search over time.
Also read Melanie from CraigScottCapital: A Leading Force in Financial Growth
Conclusion
In this article, we’ve walked through the process of building a philosophy quote generator with vector search and Astra DB (part 3). From preparing the data, setting up Astra DB, and storing vector representations of quotes to implementing a vector search system based on cosine similarity, we’ve covered everything you need to make your philosophy quote generator smarter and more efficient. build a philosophy quote generator with vector search and Astra DB (part 3) By leveraging the power of vector search and Astra DB, you can offer users an intuitive and intelligent way to explore philosophical ideas.
In the next part of our series, we will explore further optimizations and advanced techniques to refine the quote generator, making it even more powerful and scalable. Stay tuned!